Web Accessibility
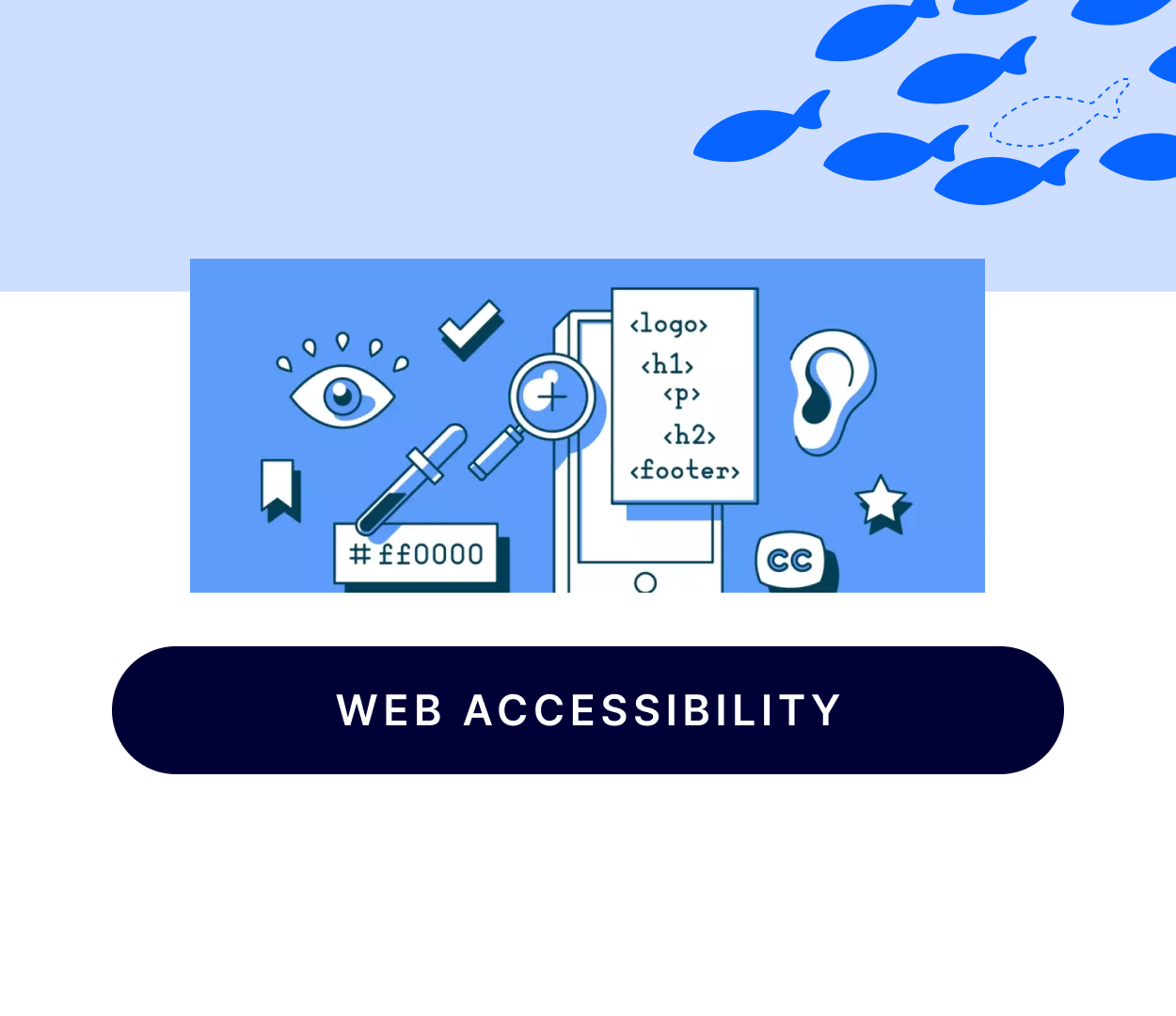
Introduction
More than a billion people (15% of the world population) have some type of motor, visual, hearing, or cognitive disability. For this reason, software applications should be adapted in such a way that everyone can use them comfortably and without limitations.
Usually, accessible websites work better for everyone. They are often faster, easier to use and appear higher in search engine rankings.
Right now, most websites meant for general use don't meet accessibility requirements.
WCAG overview
The Web Content Accessibility Guidelines defines the internationally recognized set of recommendations for making websites accessible to everyone, including users with impairments to their:
- Vision: like severely sight impaired (blind), sight impaired (partially sighted) or color blind people.
- Hearing: people who are deaf or hard of hearing.
- Mobility: those who find it difficult to use a mouse, touch screen or keyboard.
- Thinking and understanding: people with dyslexia, autism or learning difficulties.
WCAG is defined by four main principles:
Perceivable
In order to meet this principle you have to make sure all the users of the web page can recognize and use your service with the senses are available to them. So, the application has to offer alternatives for every user, for example:
- Provide transcripts for audio and video.
- Make sure the content is logically ordered and it can be navigated by assistive technologies (e.g. screen reader).
- Use contrasting colors which show up clearly against the background.
- Make sure the service is responsive, and supports different device resolutions and text sizes.
Operable
The operability principle ensures that any user can use the application regardless of how they choose to access it (for example, using a keyboard or voice commands). To achieve this, the service has to accomplish the following requirements:
- Make sure everything works for keyboard-only users.
- Let the user manage moving content as they want: pause, stop, skip or disable any animation.
- Use descriptive links, headings and labels, so users know where a link will take them, or what a title refers to.
- Make it easy for users to disable and change shortcut keys.
Understandable
Everyone should be able to understand the content of your webpage, and how the service works. To achieve that, the following ideas should be taken into account:
- Avoid using jargon, and keep sentences short and concrete.
- Provide an explanation every time abbreviations, acronyms and other words that some people could not recognize appear in the app.
- Make clear what language the content is written in, and indicate if it changes.
- Make sure all form fields have visible and meaningful labels, and that they are marked up properly.
- Make it easy for people to identify and correct any error in forms.
Robust
The last principle that WCAG defines is the robustness of the service: it should run in a wide variety of contexts, like reasonably outdated browsers and assistive technologies that the user could prefer to use.
- Use the correct HTML tags so user clients, including assistive technologies, can accurately interpret and parse the content.
- Make sure your code lets assistive technologies know what every user interface component is for, what state it is currently in and if it changes.
- Make sure important status messages or modal dialogs are marked up in a way that they inform the user of their presence and purpose, and that they let the user interact with them using assistive technology.
- Allow the user to return to what they were doing after they have interacted with the status message or modal input.
How to achieve accessibility?
The basis for an accessible website is the correct usage of the frontend development technologies.
Nowadays plenty of libraries and frameworks exist to improve performance and make the developers' life easier.
However, almost every frontend tech stack flows in the top three technologies that browsers process and understand:
HTML - CSS - JAVASCRIPT
Accessible HTML
HTML defines structure.
It is used to specify whether your web content should be recognized as a paragraph, list, heading, link, image, multimedia player, form, or one of many other available elements, or even a new element that you define.
HTML is accessible by default if it is used correctly. What does that correctness imply?
Semantic
It is fundamental to use the mentioned tags right, each for its purpose.
That means, for example, not to use <div>
tag for everything.
If you need to implement a button, just use <button>
tag, so a screen reader can reach that button, and the user can click it using Return/Enter key.
If you write a piece of code like this:
the application immediately loses the native html keyboard accessibility.
Writing semantic HTML does not take more time than doing it wrong, especially if you maintain that consistency all along the project.
Even better, the semantic HTML writing comes with benefits:
- Easier development: as it was exemplified before, some functionalities come for free.
- Works better in mobile browsers: the HTML code is probably lighter than the no-semantic spaghetti code, and it is easier to make it responsive.
- Search Engine Optimization: as it was mentioned in the introduction, search engines prioritize accessible websites. Particularly, they focus on the keywords that appear in headers (e.g:
<h1>
,<h2>
), links etc. And not in the text tagged by a<div>
.
Order
Besides having a correct semantic, HTML is the structure, so the different elements should be ordered in a way that makes sense regarding with the display order. CSS could re-order the elements to show them as it is wanted, but a coherent HTML order is fundamental to give the keyboard-only and screen reader users a good experience.
Focusing on keyboard-only users, the Tab
key comes into play.
Although the tabIndex
attribute reorders the elements to being tabulated, that option doesn’t allow to activate them using Return/Enter.
HTML forms
Another main topic to make a webpage accesible is to allow every kind of user to use forms easily.
The semantic and order (previously described) need to be taken into account when you write a form:
To begin with, the <form/>
tag needs to be used, never wrap a form in a <div/>
.
The form tag should have an action
and method
prop where you can define what happens on submit, and in order to complete that action, a type=”submit”
button is needed.
Then, the other main components in forms are the inputs
(or select
, textarea
, etc) and its corresponding label
.
HTML allows many ways to do this, but the goal here is to write an accesible form, so we need to relate the label
with its input
:
So screen readers and other tools can associate the elements and describe the form correctly.
To sum up, an example of a badly written form:
It is not recommended to use placeholder
instead of label
to describe the form's semantic, since some screen readers ignore them and the user would not know what the input is for.
A well written form would look like this:
Associating the label with the input (by for-id
) also allows the user to focus the input by clicking the label, so it makes the action area bigger.
Accesible images
The last HTML element to present are the <img/>
tags.
Nowadays most web apps use images to describe and decorate content.
If the image is merely decorative, there are two ways to write them right: they can be included as background images with CSS, or using the <img>
tag adding it the role=”presentation”
attribute, so screen readers and tab
navigation would ignore them.
On the other hand, if the image has a meaning for the web app content, an alternative description has to be always provided. In case the image is not available or the user navigates with a screen reader tool that description would be shown.
The common way to do it is using the alt
prop, which should provide a direct representation of the image and what it visually transmits.
Also, the image description can be added using a regular <p>
tag, and defining the aria-labelledby
attribute in the <img>
with the same value as the <p>
id
. This way, more than one image can be related to the same description.
Accessible CSS
The style of a web application is fundamental to make it so everyone can use it comfortably.
- Select reasonable font sizes, line heights, letter spacing, etc. so the text styling is coherent with each role, and comfortable to read. For example, a title should be differentiated from the body text, and a list should look like one.
- Make it responsive. The page has to support larger text sizes for users who wish to use them. And the content should not be hidden on any screen size.
- The color of text and buttons must contrast with the background. There are contrast verification tools. Besides helping low vision users, every person benefits from this, for example when they use their phone or tablet under sunlight.
- The text color should be coherent with its role, for example,
errors
usually are displayed in red color so the user understands that something is wrong. Alsoplaceholders
should have a lighter color than the rest of the text. - Associate the right cursor type for each case for a better comprehension. For example:
cursor: pointer
for buttons, andcursor: not-allowed
on disabled.
Accessible Javascript
JavaScript can also break accessibility, depending on how it is used.
Modern JavaScript is a powerful language, and we can do so much with it these days, but you should make your JavaScript non-intrusive when creating your content. The idea of non-intrusive JavaScript is that it should be used wherever possible to enhance functionality, not to build everything; the basic functions work ideally without JavaScript, that is what HTML is for.
A big accessibility part is to use the browser built-in features whenever possible.
Two main points to consider are the mouse events and the error displaying. Some of the mouse events are not compatible with alternative ways to use the web page, like keyboard-only. For example, mouseover
, mouseout
, dblclick
, actions cannot be activated by keyboard.
In order to mitigate this problem, you should duplicate those events with the ones that are accesible by alternative usage mechanisms, called device-independent event handlers. For example, focus
and blur
would provide accessibility for keyboard-only users.
On the other hand, error handling is very important for accessibility. It is fundamental to give descriptive error messages, and link each one with the correct element (e.g. input) where the error was created.
Given a form, with different items, and a createLink()
function which relates the error with the corresponding item, this would be the validation function for no empty inputs:
In this example, we are hiding and showing the error message box using absolute positioning rather than another CSS method such as visibility
or display
, because it does not interfere with the screen reader being able to read content from it.
Conclusion
Remember, accessibility starts from the beginning, with the user interface designs and the code architecture.
Accessibility benefits everyone: the app owner, developers, and every kind of user. Websites that ignore accessibility exclude a segment of the population that stands to gain the most from the internet. As you become aware of, and correctly implement, accessibility, you can do your part to ensure the web can be used by a broader population.
So, go ahead and make your web app comfortably navigable to everyone.